
- Send your Feedback to [email protected]
Help Others, Please Share

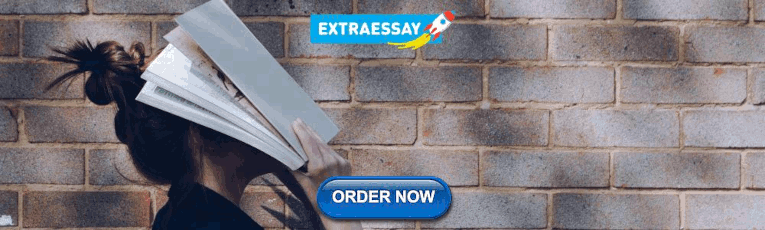
Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
- Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
- Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
Java class and objects.
- Java Methods
- Java Method Overloading
- Java Constructors
Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
Java Singleton Class
- Java enum Constructor
- Java enum Strings
Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
- Java Operator Precedence
- Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
Java is an object-oriented programming language. The core concept of the object-oriented approach is to break complex problems into smaller objects.
An object is any entity that has a state and behavior . For example, a bicycle is an object. It has
- States : idle, first gear, etc
- Behaviors : braking, accelerating, etc.
Before we learn about objects, let's first know about classes in Java.
A class is a blueprint for the object. Before we create an object, we first need to define the class.
We can think of the class as a sketch (prototype) of a house. It contains all the details about the floors, doors, windows, etc. Based on these descriptions we build the house. House is the object.
Since many houses can be made from the same description, we can create many objects from a class.
We can create a class in Java using the class keyword. For example,
Here, fields ( variables ) and methods represent the state and behavior of the object respectively.
- fields are used to store data
- methods are used to perform some operations
For our bicycle object, we can create the class as
In the above example, we have created a class named Bicycle . It contains a field named gear and a method named braking() .
Here, Bicycle is a prototype. Now, we can create any number of bicycles using the prototype. And, all the bicycles will share the fields and methods of the prototype.
Note : We have used keywords private and public . These are known as access modifiers. To learn more, visit Java access modifiers .
An object is called an instance of a class. For example, suppose Bicycle is a class then MountainBicycle , SportsBicycle , TouringBicycle , etc can be considered as objects of the class.
Creating an Object in Java
Here is how we can create an object of a class.
We have used the new keyword along with the constructor of the class to create an object. Constructors are similar to methods and have the same name as the class. For example, Bicycle() is the constructor of the Bicycle class. To learn more, visit Java Constructors .
Here, sportsBicycle and touringBicycle are the names of objects. We can use them to access fields and methods of the class.
As you can see, we have created two objects of the class. We can create multiple objects of a single class in Java.
Note : Fields and methods of a class are also called members of the class.
- Access Members of a Class
We can use the name of objects along with the . operator to access members of a class. For example,
In the above example, we have created a class named Bicycle . It includes a field named gear and a method named braking() . Notice the statement,
Here, we have created an object of Bicycle named sportsBicycle . We then use the object to access the field and method of the class.
- sportsBicycle.gear - access the field gear
- sportsBicycle.braking() - access the method braking()
We have mentioned the word method quite a few times. You will learn about Java methods in detail in the next chapter.
Now that we understand what is class and object. Let's see a fully working example.
- Example: Java Class and Objects
In the above program, we have created a class named Lamp . It contains a variable: isOn and two methods: turnOn() and turnOff() .
Inside the Main class, we have created two objects: led and halogen of the Lamp class. We then used the objects to call the methods of the class.
- led.turnOn() - It sets the isOn variable to true and prints the output.
- halogen.turnOff() - It sets the isOn variable to false and prints the output.
The variable isOn defined inside the class is also called an instance variable. It is because when we create an object of the class, it is called an instance of the class. And, each instance will have its own copy of the variable.
That is, led and halogen objects will have their own copy of the isOn variable.
Example: Create objects inside the same class
Note that in the previous example, we have created objects inside another class and accessed the members from that class.
However, we can also create objects inside the same class.
Here, we are creating the object inside the main() method of the same class.
Table of Contents
- Introduction
- Example: Create objects inside a class
Sorry about that.
Related Tutorials
Java Tutorial
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Tutorial
Overview of Java
- Introduction to Java
- The Complete History of Java Programming Language
- C++ vs Java vs Python
- How to Download and Install Java for 64 bit machine?
- Setting up the environment in Java
- How to Download and Install Eclipse on Windows?
- JDK in Java
- How JVM Works - JVM Architecture?
- Differences between JDK, JRE and JVM
- Just In Time Compiler
- Difference between JIT and JVM in Java
- Difference between Byte Code and Machine Code
- How is Java platform independent?
Basics of Java
- Java Basic Syntax
- Java Hello World Program
- Java Data Types
- Primitive data type vs. Object data type in Java with Examples
- Java Identifiers
Operators in Java
- Java Variables
- Scope of Variables In Java
Wrapper Classes in Java
Input/output in java.
- How to Take Input From User in Java?
- Scanner Class in Java
- Java.io.BufferedReader Class in Java
- Difference Between Scanner and BufferedReader Class in Java
- Ways to read input from console in Java
- System.out.println in Java
- Difference between print() and println() in Java
- Formatted Output in Java using printf()
- Fast I/O in Java in Competitive Programming
Flow Control in Java
- Decision Making in Java (if, if-else, switch, break, continue, jump)
- Java if statement with Examples
- Java if-else
- Java if-else-if ladder with Examples
- Loops in Java
- For Loop in Java
- Java while loop with Examples
- Java do-while loop with Examples
- For-each loop in Java
- Continue Statement in Java
- Break statement in Java
- Usage of Break keyword in Java
- return keyword in Java
- Java Arithmetic Operators with Examples
- Java Unary Operator with Examples
Java Assignment Operators with Examples
- Java Relational Operators with Examples
- Java Logical Operators with Examples
- Java Ternary Operator with Examples
- Bitwise Operators in Java
- Strings in Java
- String class in Java
- Java.lang.String class in Java | Set 2
- Why Java Strings are Immutable?
- StringBuffer class in Java
- StringBuilder Class in Java with Examples
- String vs StringBuilder vs StringBuffer in Java
- StringTokenizer Class in Java
- StringTokenizer Methods in Java with Examples | Set 2
- StringJoiner Class in Java
- Arrays in Java
- Arrays class in Java
- Multidimensional Arrays in Java
- Different Ways To Declare And Initialize 2-D Array in Java
- Jagged Array in Java
- Final Arrays in Java
- Reflection Array Class in Java
- util.Arrays vs reflect.Array in Java with Examples
OOPS in Java
- Object Oriented Programming (OOPs) Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects in Java
- Naming Conventions in Java
- Java Methods
Access Modifiers in Java
- Java Constructors
- Four Main Object Oriented Programming Concepts of Java
Inheritance in Java
Abstraction in java, encapsulation in java, polymorphism in java, interfaces in java.
- 'this' reference in Java
- Inheritance and Constructors in Java
- Java and Multiple Inheritance
- Interfaces and Inheritance in Java
- Association, Composition and Aggregation in Java
- Comparison of Inheritance in C++ and Java
- abstract keyword in java
- Abstract Class in Java
- Difference between Abstract Class and Interface in Java
- Control Abstraction in Java with Examples
- Difference Between Data Hiding and Abstraction in Java
- Difference between Abstraction and Encapsulation in Java with Examples
- Difference between Inheritance and Polymorphism
- Dynamic Method Dispatch or Runtime Polymorphism in Java
- Difference between Compile-time and Run-time Polymorphism in Java
Constructors in Java
- Copy Constructor in Java
- Constructor Overloading in Java
- Constructor Chaining In Java with Examples
- Private Constructors and Singleton Classes in Java
Methods in Java
- Static methods vs Instance methods in Java
- Abstract Method in Java with Examples
- Overriding in Java
- Method Overloading in Java
- Difference Between Method Overloading and Method Overriding in Java
- Differences between Interface and Class in Java
- Functional Interfaces in Java
- Nested Interface in Java
- Marker interface in Java
- Comparator Interface in Java with Examples
- Need of Wrapper Classes in Java
- Different Ways to Create the Instances of Wrapper Classes in Java
- Character Class in Java
- Java.Lang.Byte class in Java
- Java.Lang.Short class in Java
- Java.lang.Integer class in Java
- Java.Lang.Long class in Java
- Java.Lang.Float class in Java
- Java.Lang.Double Class in Java
- Java.lang.Boolean Class in Java
- Autoboxing and Unboxing in Java
- Type conversion in Java with Examples
Keywords in Java
- Java Keywords
- Important Keywords in Java
- Super Keyword in Java
- final Keyword in Java
- static Keyword in Java
- enum in Java
- transient keyword in Java
- volatile Keyword in Java
- final, finally and finalize in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access and Non Access Modifiers in Java
Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory?
- Stack vs Heap Memory Allocation
- How many types of memory areas are allocated by JVM?
- Garbage Collection in Java
- Types of JVM Garbage Collectors in Java with implementation details
- Memory leaks in Java
- Java Virtual Machine (JVM) Stack Area
Classes of Java
- Understanding Classes and Objects in Java
- Singleton Method Design Pattern in Java
- Object Class in Java
- Inner Class in Java
- Throwable Class in Java with Examples
Packages in Java
- Packages In Java
- How to Create a Package in Java?
- Java.util Package in Java
- Java.lang package in Java
- Java.io Package in Java
- Java Collection Tutorial
Exception Handling in Java
- Exceptions in Java
- Types of Exception in Java with Examples
- Checked vs Unchecked Exceptions in Java
- Java Try Catch Block
- Flow control in try catch finally in Java
- throw and throws in Java
- User-defined Custom Exception in Java
- Chained Exceptions in Java
- Null Pointer Exception In Java
- Exception Handling with Method Overriding in Java
- Multithreading in Java
- Lifecycle and States of a Thread in Java
- Java Thread Priority in Multithreading
- Main thread in Java
- Java.lang.Thread Class in Java
- Runnable interface in Java
- Naming a thread and fetching name of current thread in Java
- What does start() function do in multithreading in Java?
- Difference between Thread.start() and Thread.run() in Java
- Thread.sleep() Method in Java With Examples
- Synchronization in Java
- Importance of Thread Synchronization in Java
- Method and Block Synchronization in Java
- Lock framework vs Thread synchronization in Java
- Difference Between Atomic, Volatile and Synchronized in Java
- Deadlock in Java Multithreading
- Deadlock Prevention And Avoidance
- Difference Between Lock and Monitor in Java Concurrency
- Reentrant Lock in Java
File Handling in Java
- Java.io.File Class in Java
- Java Program to Create a New File
- Different ways of Reading a text file in Java
- Java Program to Write into a File
- Delete a File Using Java
- File Permissions in Java
- FileWriter Class in Java
- Java.io.FileDescriptor in Java
- Java.io.RandomAccessFile Class Method | Set 1
- Regular Expressions in Java
- Regex Tutorial - How to write Regular Expressions?
- Matcher pattern() method in Java with Examples
- Pattern pattern() method in Java with Examples
- Quantifiers in Java
- java.lang.Character class methods | Set 1
- Java IO : Input-output in Java with Examples
- Java.io.Reader class in Java
- Java.io.Writer Class in Java
- Java.io.FileInputStream Class in Java
- FileOutputStream in Java
- Java.io.BufferedOutputStream class in Java
- Java Networking
- TCP/IP Model
- User Datagram Protocol (UDP)
- Difference Between IPv4 and IPv6
- Difference between Connection-oriented and Connection-less Services
- Socket Programming in Java
- java.net.ServerSocket Class in Java
- URL Class in Java with Examples
JDBC - Java Database Connectivity
- Introduction to JDBC (Java Database Connectivity)
- JDBC Drivers
- Establishing JDBC Connection in Java
- Types of Statements in JDBC
- JDBC Tutorial
- Java 8 Features - Complete Tutorial
Operators constitute the basic building block of any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they provide.
Types of Operators:
- Arithmetic Operators
- Unary Operators
- Assignment Operator
- Relational Operators
- Logical Operators
- Ternary Operator
- Bitwise Operators
- Shift Operators
This article explains all that one needs to know regarding Assignment Operators.
Assignment Operators
These operators are used to assign values to a variable. The left side operand of the assignment operator is a variable, and the right side operand of the assignment operator is a value. The value on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. This means that the assignment operators have right to left associativity, i.e., the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side value must be declared before using it or should be a constant. The general format of the assignment operator is,
Types of Assignment Operators in Java
The Assignment Operator is generally of two types. They are:
1. Simple Assignment Operator: The Simple Assignment Operator is used with the “=” sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
2. Compound Assignment Operator: The Compound Operator is used where +,-,*, and / is used along with the = operator.
Let’s look at each of the assignment operators and how they operate:
1. (=) operator:
This is the most straightforward assignment operator, which is used to assign the value on the right to the variable on the left. This is the basic definition of an assignment operator and how it functions.
Syntax:
Example:
2. (+=) operator:
This operator is a compound of ‘+’ and ‘=’ operators. It operates by adding the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
Note: The compound assignment operator in Java performs implicit type casting. Let’s consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5 Method 2: x += 4.5 As per the previous example, you might think both of them are equal. But in reality, Method 1 will throw a runtime error stating the “i ncompatible types: possible lossy conversion from double to int “, Method 2 will run without any error and prints 9 as output.
Reason for the Above Calculation
Method 1 will result in a runtime error stating “incompatible types: possible lossy conversion from double to int.” The reason is that the addition of an int and a double results in a double value. Assigning this double value back to the int variable x requires an explicit type casting because it may result in a loss of precision. Without the explicit cast, the compiler throws an error. Method 2 will run without any error and print the value 9 as output. The compound assignment operator += performs an implicit type conversion, also known as an automatic narrowing primitive conversion from double to int . It is equivalent to x = (int) (x + 4.5) , where the result of the addition is explicitly cast to an int . The fractional part of the double value is truncated, and the resulting int value is assigned back to x . It is advisable to use Method 2 ( x += 4.5 ) to avoid runtime errors and to obtain the desired output.
Same automatic narrowing primitive conversion is applicable for other compound assignment operators as well, including -= , *= , /= , and %= .
3. (-=) operator:
This operator is a compound of ‘-‘ and ‘=’ operators. It operates by subtracting the variable’s value on the right from the current value of the variable on the left and then assigning the result to the operand on the left.
4. (*=) operator:
This operator is a compound of ‘*’ and ‘=’ operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
5. (/=) operator:
This operator is a compound of ‘/’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the quotient to the operand on the left.
6. (%=) operator:
This operator is a compound of ‘%’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the remainder to the operand on the left.
Please Login to comment...
Similar reads.
Improve your Coding Skills with Practice

What kind of Experience do you want to share?
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
JavaScript ( JS ) is a lightweight interpreted (or just-in-time compiled) programming language with first-class functions . While it is most well-known as the scripting language for Web pages, many non-browser environments also use it, such as Node.js , Apache CouchDB and Adobe Acrobat . JavaScript is a prototype-based , multi-paradigm, single-threaded , dynamic language, supporting object-oriented, imperative, and declarative (e.g. functional programming) styles.
JavaScript's dynamic capabilities include runtime object construction, variable parameter lists, function variables, dynamic script creation (via eval ), object introspection (via for...in and Object utilities ), and source-code recovery (JavaScript functions store their source text and can be retrieved through toString() ).
This section is dedicated to the JavaScript language itself, and not the parts that are specific to Web pages or other host environments. For information about APIs that are specific to Web pages, please see Web APIs and DOM .
The standards for JavaScript are the ECMAScript Language Specification (ECMA-262) and the ECMAScript Internationalization API specification (ECMA-402). As soon as one browser implements a feature, we try to document it. This means that cases where some proposals for new ECMAScript features have already been implemented in browsers, documentation and examples in MDN articles may use some of those new features. Most of the time, this happens between the stages 3 and 4, and is usually before the spec is officially published.
Do not confuse JavaScript with the Java programming language — JavaScript is not "Interpreted Java" . Both "Java" and "JavaScript" are trademarks or registered trademarks of Oracle in the U.S. and other countries. However, the two programming languages have very different syntax, semantics, and use.
JavaScript documentation of core language features (pure ECMAScript , for the most part) includes the following:
- The JavaScript guide
- The JavaScript reference
For more information about JavaScript specifications and related technologies, see JavaScript technologies overview .
Learn how to program in JavaScript with guides and tutorials.
For complete beginners
Head over to our Learning Area JavaScript topic if you want to learn JavaScript but have no previous experience with JavaScript or programming. The complete modules available there are as follows:
Answers some fundamental questions such as "what is JavaScript?", "what does it look like?", and "what can it do?", along with discussing key JavaScript features such as variables, strings, numbers, and arrays.
Continues our coverage of JavaScript's key fundamental features, turning our attention to commonly-encountered types of code blocks such as conditional statements, loops, functions, and events.
The object-oriented nature of JavaScript is important to understand if you want to go further with your knowledge of the language and write more efficient code, therefore we've provided this module to help you.
Discusses asynchronous JavaScript, why it is important, and how it can be used to effectively handle potential blocking operations such as fetching resources from a server.
Explores what APIs are, and how to use some of the most common APIs you'll come across often in your development work.
JavaScript guide
A much more detailed guide to the JavaScript language, aimed at those with previous programming experience either in JavaScript or another language.
Intermediate
JavaScript frameworks are an essential part of modern front-end web development, providing developers with proven tools for building scalable, interactive web applications. This module gives you some fundamental background knowledge about how client-side frameworks work and how they fit into your toolset, before moving on to a series of tutorials covering some of today's most popular ones.
An overview of the basic syntax and semantics of JavaScript for those coming from other programming languages to get up to speed.
Overview of available data structures in JavaScript.
JavaScript provides three different value comparison operations: strict equality using === , loose equality using == , and the Object.is() method.
How different methods that visit a group of object properties one-by-one handle the enumerability and ownership of properties.
A closure is the combination of a function and the lexical environment within which that function was declared.
Explanation of the widely misunderstood and underestimated prototype-based inheritance.
Memory life cycle and garbage collection in JavaScript.
JavaScript has a runtime model based on an "event loop".
Browse the complete JavaScript reference documentation.
Get to know standard built-in objects Array , Boolean , Date , Error , Function , JSON , Math , Number , Object , RegExp , String , Map , Set , WeakMap , WeakSet , and others.
Learn more about the behavior of JavaScript's operators instanceof , typeof , new , this , the operator precedence , and more.
Learn how do-while , for-in , for-of , try-catch , let , var , const , if-else , switch , and more JavaScript statements and keywords work.
Learn how to work with JavaScript's functions to develop your applications.
JavaScript classes are the most appropriate way to do object-oriented programming.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Why is reference assignment atomic in Java?
As far as I know reference assignment is atomic in a 64 bit JVM. Now, I assume the jvm doesn't use atomic pointers internally to model this, since otherwise there would be no need for Atomic References. So my questions are:
Is atomic reference assignment in the "specs" of java/Scala and guaranteed to happen or is it just a happy coincidence that it is that way most times ?
Is atomic reference assignment implied for any language that compiles to the JVM's bytecode (e.g. clojure, Groovy, JRuby, JPython...etc) ?
How can reference assignment be atomic without using an atomic pointer internally ?

- @JornVernee I meant assignment not swapping, thanks for the correction – George Commented May 31, 2017 at 11:21
- This might give a first clue: stackoverflow.com/questions/23232242/… – GhostCat Commented May 31, 2017 at 11:35
- I did... however I've been searching all over the internet for how getfield, putfiled and iastore are implemented and I haven't found an explaination that doesn't involve digging through the openjvm code, which I'm not proficient enough to do :( – George Commented May 31, 2017 at 12:23
3 Answers 3
First of all, reference assignment is atomic because the specification says so. Besides that, there is no obstacle for JVM implementors to fulfill this constraint, as 64 Bit references are usually only used on 64 Bit architectures, where atomic 64 Bit assignment comes for free.
Your main confusion stems from the assumption that the additional “Atomic References” feature means exactly that, due to its name. But the AtomicReference class offers far more, as it encapsulates a volatile reference, which has stronger memory visibility guarantees in a multi-threaded execution.
Having an atomic reference update does not necessarily imply that a thread reading the reference will also see consistent values regarding the fields of the object reachable through that reference. All it guarantees is that you will read either the null reference or a valid reference to an existing object that actually was stored by some thread. If you want more guarantees, you need constructs like synchronization, volatile references, or an AtomicReference .
AtomicReference also offers atomic update operations like compareAndSet or getAndSet . These are not possible with ordinary reference variables using built-in language constructs (but only with special classes like AtomicReferenceFieldUpdater or VarHandle ).

- 2 An “atomic pointer” isn’t necessarily an “atomic reference”, in the sense of AtomicReference . Hence, it was necessary to point out that references (i.e. the underlying pointers) are atomic, still don’t match the functionality of an AtomicReference . – Holger Commented May 31, 2017 at 15:54
- 3 “Publishing” means assigning to a variable that is visible to other threads. And no, there are no guaranties regarding visibility of previous updates when publishing though normal references. If you write point=new Point(42,100) and point is neither final nor volatile , other threads may see the new reference, while still seeing the default value ( 0 ) for either, x or y , or both (in the absence of other synchronization). – Holger Commented May 31, 2017 at 15:57
- 3 This is not about an older instance; the reference might have been null before the assignment, that doesn’t matter. The issue is, new Point(42,100) is not an atomic operation. It creates a new Point instance, all fields being at their default value ( 0 for int ), then, the constructor will be executed, which will assign the values 42 to x and 100 to y , then the reference is assigned to point , from a single thread’s point of view . A different thread may see these actions out of order, see the reference to the new Point instance without seeing the effect of the assignments. – Holger Commented May 31, 2017 at 16:36
- 2 This doesn’t apply to immutable objects. If you declare x and y as final , they’re immune to such data races. Alternatively, you may declare point as final , but this implies that the whole action is part of a constructor or initializer. Or, well, declaring point as volatile ensures that other threads see all previous updates ( x=42; y=100; ) when seeing the new Point instance through the point reference. – Holger Commented May 31, 2017 at 16:37
- 2 @Powet any reference assignment that doesn’t care about the previous reference, is atomic. The update operations provided by AtomicReference include compareAndSet (“only assign the new reference if the old reference has the expected value”) and getAndSet (“give me the old reference after assigning the new one”). If you try to implement the same logic without AtomicReference , it will inevitably consist of more than one access to the field, so it’ll be obvious why this wouldn’t be atomic (without additional measures). – Holger Commented Apr 16 at 8:16
Atomic reference assignment is in the specs.
Writes to and reads of references are always atomic, regardless of whether they are implemented as 32 or 64 bit values.
Quoted from JSR-133: Java(TM) Memory Model and Thread Specification , section 12 Non-atomic Treatment of double and long , http://www.cs.umd.edu/~pugh/java/memoryModel/jsr133.pdf .

- Ok, that answers the first part but not the other 2. To be honest the thing that I'm most curios about here is how this behavior happens if references are not atomic pointers and that's related to how one would implement a JVM rather than the specifications for java and/or the jvm. – George Commented May 31, 2017 at 12:17
- True. I on purpose did not pretend to be a wiseguy on matters where I’m not sure. The way I understand it, though, this is a spec of the JVM, so I would expect it to cover all languages running on the JVM. And the way I read it, in requires internal pointer operations to happen atomically (at least as seen from outside the JVM). – Anonymous Commented May 31, 2017 at 12:25
- Well yes, but I doubt those are actual atomic pointer operations since otherwise things like compare and swap would also be atomic using standard references. – George Commented May 31, 2017 at 12:29
- 1 Nice answer - good find; my vote for that. And thanks for leaving out that small part that left room for my answer ;-) – GhostCat Commented May 31, 2017 at 12:29
- 3 The linked PDF describes the memory model for Java 5 proposed by JSR133. To prove that it indeed made it into the Java 5 JLS and is still there today, it’s better to link to JLS §17.7 . – Holger Commented May 31, 2017 at 14:38
As the other answer outlines, the Java Memory Model states that references read/writes are atomic.
But of course, that is the Java language memory model. On the other hand: no matter if we talk Java or Scala or Kotlin or ... in the end everything gets compiled into bytecode .
There are no special bytecode instructions for Java. Scala in the end uses the very same instructions.
Leading to: the properties of that memory model must be implemented inside the VM platform. Thus they must apply to other languages running on the platform as well.

- Since the name was ' JavaTM Memory Model' I was unsure if it referred to the JVM as a whole and as such I assumed there might be instructions to non atomically asign available to other JVM languages – George Commented May 31, 2017 at 12:40
- Back then when that memory model was written, there was only the Java language on the Java VM platform. Methinks. – GhostCat Commented May 31, 2017 at 12:41
- Even now, I don't think there are JVM instructions that aren't used by Java itself. – Jasper-M Commented May 31, 2017 at 12:44
- 5 @Jasper-M: there are. E.g., swap is never used by javac generated code. In Java 7, the invokedynamic instruction was not used by Java itself, which is quite a big feature of that JVM version… – Holger Commented May 31, 2017 at 14:26
- 3 One thing to keep in mind when drawing this conclusion, is, that this only applies if the concepts of that other language (i.e. references) are compiled 1:1 to the equivalent byte code artifacts. E.g. a reference of a particular language might be so far away from what Java’s object references do, that it doesn’t match a sole byte code reference variable, but, e.g. a compound data structure. For Java, it is impossible to encounter an object reference having a not-yet-initialized vtable, but that doesn’t have to apply to another language, having an entirely different method dispatch algorithm. – Holger Commented Jun 1, 2017 at 8:28
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged java scala concurrency jvm atomic or ask your own question .
- Featured on Meta
- Announcing a change to the data-dump process
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
Hot Network Questions
- What to do about chain rubbing on unusually placed chainstay?
- Where can I find a switch to alternate connections between two pairs of two wires?
- Report a police emergency at home while abroad
- UK citizen travel document to enter Ireland by air
- What are the not-winglet wingtips on the CL-415?
- Bicolor/double-sided feathers as part of a thermoregulatory apparatus?
- Connect 3 on different board sizes
- 2008 civic ex where do wires from connector on top of alternator run to
- Which Jesus died or in what sense did Jesus ("God") die for our sins
- Are "very alone" and "very much alone" interchangeable?
- Can someone legally go out in public with only their underwear on?
- Structure of the headline "Olympics organizers scramble as furor over woke blasphemy grows."
- What do the H and the E stand for in John Cohn's name?
- Is there a difference between "set" and "collection"?
- How do manganese nodules in the ocean sustain oxygen production without depleting over geological time scales?
- Why try to explain the unexplainable?
- What concerns are there with soldering stainless steel cable on electronics?
- Is it dangerous if an LED lightbulb fails to light up?
- Array of Objects not getting reflecting in LWC html
- Pistorius: “We must be ready for war by 2029”. Why 2029?
- Terminology: A "corollary" to a proof?
- What did Rohan and Gondor think Sauron was?
- Why is it plural for one example and singular for the second
- How can life which cannot live on the surface of a planet naturally reach the supermajority of the planet's caves?

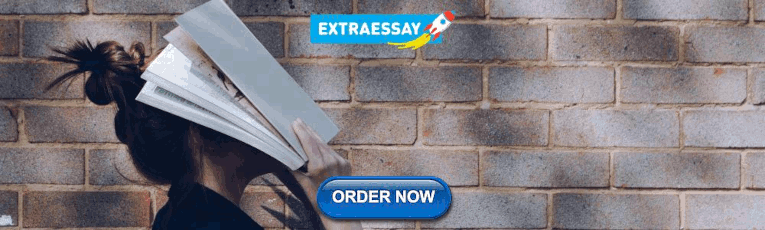
IMAGES
VIDEO
COMMENTS
Test t2 = t1; You are actually creating another Test reference, and you assign it to refer to the same object that t1 refers to. So t1.i = 1; will affect t2.i for it is the same object after all. As for the Strings, Strings are immutable and can not be modified after instantiated. Regarding your edit:
1. Reference variable is used to point object/values. 2. Classes, interfaces, arrays, enumerations, and, annotations are reference types in Java. Reference variables hold the objects/values of reference types in Java. 3. Reference variable can also store null value. By default, if no object is passed to a reference variable then it will store a ...
The first statement invokes rectOne's getArea() method and displays the results. The second line moves rectTwo because the move() method assigns new values to the object's origin.x and origin.y.. As with instance fields, objectReference must be a reference to an object. You can use a variable name, but you also can use any expression that returns an object reference.
By the way, all Java objects are polymorphic because each object is an Object at least. We can assign an instance of Animal to the reference variable of Object type and the compiler won't complain: Object object = new Animal(); That's why all Java objects we create already have Object-specific methods, for example toString().
Object reference variables act differently when an assignment takes place. For example, Box b1 = new Box(); Box b2 = b1; After this fragment executes, b1 and b2 will both refer to the same object. The assignment of b1 to b2 did not allocate any memory or copy any part of the original object. It simply makes b2 refer to the same object as does b1.
The object is unreferenced, and its resources are free to be recycled by the Java Virtual Machine. Calling an Object's Methods. You also use an object reference to invoke an object's method. You append the method's simple name to the object reference, with an intervening dot operator (.). Also, you provide, within enclosing parentheses, any ...
Dereferencing. Dereferencing: Accessing the value of the pointee for some reference variable. Is done with the "dot" (.) operator to access a field or method of an object. Examples: dereferencing empRef in the previous slide gives back its pointee, the Employee object. String myName = empRef.getName();
2. How does the assignment of objects = is realized? General form. The assignment operation has the following general form: obj1 = obj2; here. obj1, obj2 - reference to objects that have the same type (class). When assigning objects, the value of the reference to the object obj2 is assigned to the reference obj1. In fact, only references are ...
Reference Variables and Assignment. In an assignment operation, object reference variables act differently than do variables of a primitive type, such as int. When you assign one primitive-type variable to another, the situation is straightforward. The variable on the left receives a copy of the value of the variable on the right.
Compound Assignment Operators. Sometime we need to modify the same variable value and reassigned it to a same reference variable. Java allows you to combine assignment and addition operators using a shorthand operator. For example, the preceding statement can be written as: i +=8; //This is same as i = i+8; The += is called the addition ...
Lesson 10- Object References. INTRODUCTION: This lesson discusses object reference variables. These variables refer to objects (as opposed to holding a primitive data value.) The key topics for this lesson are: A. Primitive Data Type Variables. B. Object Reference variables. C. Variable Versus Object Reference Assignment.
In Java, we create Classes. We create objects of the Classes. Let us know if it is possible to assign a Java Object from one variable to another variable by value or reference in this Last Minute Java Tutorial. We also try to know whether it is possible to pass Java Objects to another method by Value or Reference in this tutorial.. You may also read about Introduction to Java Class Structure.
Passing by value means we pass a copy of the value.; Passing by reference means we pass the real reference to the variable in memory.; Java object references are passed by value. All object ...
In Java, a reference variable is a variable that holds the memory address of an object rather than the actual object itself. It acts as a reference to the object and allows manipulation of its data and methods. Reference variables are declared with a specific type, which determines the methods and fields that can be accessed through that ...
Java Class and Objects. Java is an object-oriented programming language. The core concept of the object-oriented approach is to break complex problems into smaller objects. An object is any entity that has a state and behavior. For example, a bicycle is an object. It has. States: idle, first gear, etc. Behaviors: braking, accelerating, etc.
Object obj1=Object() Object obj2=obj1; two objects will be created. That is property values of obj1 are copied to obj2. If you do not want to create a new object, you should use pointers. Ex: Object obj1=Object() Object *obj1ref=&obj1; //this is like assigning reference in java.
Note: The compound assignment operator in Java performs implicit type casting. Let's consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5. Method 2: x += 4.5.
JavaScript (JS) is a lightweight interpreted (or just-in-time compiled) programming language with first-class functions. While it is most well-known as the scripting language for Web pages, many non-browser environments also use it, such as Node.js, Apache CouchDB and Adobe Acrobat. JavaScript is a prototype-based, multi-paradigm, single-threaded, dynamic language, supporting object-oriented ...
Like all Java objects, arrays are passed by value ... but the value is the reference to the array. So, when you assign something to a cell of the array in the called method, you will be assigning to the same array object that the caller sees. This is NOT pass-by-reference. Real pass-by-reference involves passing the address of a variable.
First of all, reference assignment is atomic because the specification says so. Besides that, there is no obstacle for JVM implementors to fulfill this constraint, as 64 Bit references are usually only used on 64 Bit architectures, where atomic 64 Bit assignment comes for free. Your main confusion stems from the assumption that the additional ...