Java Tutorial
Java methods, java classes, java file handling, java how to's, java reference, java examples, java operators.
Operators are used to perform operations on variables and values.
In the example below, we use the + operator to add together two values:
Try it Yourself »
Although the + operator is often used to add together two values, like in the example above, it can also be used to add together a variable and a value, or a variable and another variable:
Java divides the operators into the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- Bitwise operators
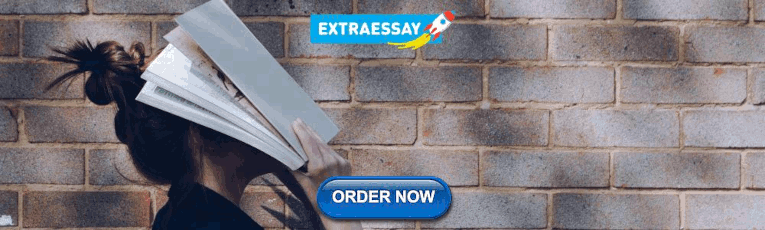
Arithmetic Operators
Arithmetic operators are used to perform common mathematical operations.
Advertisement
Java Assignment Operators
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Java Comparison Operators
Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions.
The return value of a comparison is either true or false . These values are known as Boolean values , and you will learn more about them in the Booleans and If..Else chapter.
In the following example, we use the greater than operator ( > ) to find out if 5 is greater than 3:
Java Logical Operators
You can also test for true or false values with logical operators.
Logical operators are used to determine the logic between variables or values:
Java Bitwise Operators
Bitwise operators are used to perform binary logic with the bits of an integer or long integer.
Note: The Bitwise examples above use 4-bit unsigned examples, but Java uses 32-bit signed integers and 64-bit signed long integers. Because of this, in Java, ~5 will not return 10. It will return -6. ~00000000000000000000000000000101 will return 11111111111111111111111111111010
In Java, 9 >> 1 will not return 12. It will return 4. 00000000000000000000000000001001 >> 1 will return 00000000000000000000000000000100

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, certification courses.
Created with over a decade of experience and thousands of feedback.
Java Introduction
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
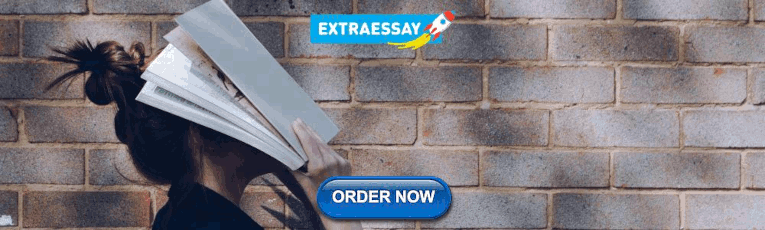
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
Java Operator Precedence
Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
- Java Math IEEEremainder()
Operators are symbols that perform operations on variables and values. For example, + is an operator used for addition, while * is also an operator used for multiplication.
Operators in Java can be classified into 5 types:
- Arithmetic Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- Unary Operators
- Bitwise Operators
1. Java Arithmetic Operators
Arithmetic operators are used to perform arithmetic operations on variables and data. For example,
Here, the + operator is used to add two variables a and b . Similarly, there are various other arithmetic operators in Java.
Example 1: Arithmetic Operators
In the above example, we have used + , - , and * operators to compute addition, subtraction, and multiplication operations.
/ Division Operator
Note the operation, a / b in our program. The / operator is the division operator.
If we use the division operator with two integers, then the resulting quotient will also be an integer. And, if one of the operands is a floating-point number, we will get the result will also be in floating-point.
% Modulo Operator
The modulo operator % computes the remainder. When a = 7 is divided by b = 4 , the remainder is 3 .
Note : The % operator is mainly used with integers.
2. Java Assignment Operators
Assignment operators are used in Java to assign values to variables. For example,
Here, = is the assignment operator. It assigns the value on its right to the variable on its left. That is, 5 is assigned to the variable age .
Let's see some more assignment operators available in Java.
Example 2: Assignment Operators
3. java relational operators.
Relational operators are used to check the relationship between two operands. For example,
Here, < operator is the relational operator. It checks if a is less than b or not.
It returns either true or false .
Example 3: Relational Operators
Note : Relational operators are used in decision making and loops.
4. Java Logical Operators
Logical operators are used to check whether an expression is true or false . They are used in decision making.
Example 4: Logical Operators
Working of Program
- (5 > 3) && (8 > 5) returns true because both (5 > 3) and (8 > 5) are true .
- (5 > 3) && (8 < 5) returns false because the expression (8 < 5) is false .
- (5 < 3) || (8 > 5) returns true because the expression (8 > 5) is true .
- (5 > 3) || (8 < 5) returns true because the expression (5 > 3) is true .
- (5 < 3) || (8 < 5) returns false because both (5 < 3) and (8 < 5) are false .
- !(5 == 3) returns true because 5 == 3 is false .
- !(5 > 3) returns false because 5 > 3 is true .
5. Java Unary Operators
Unary operators are used with only one operand. For example, ++ is a unary operator that increases the value of a variable by 1 . That is, ++5 will return 6 .
Different types of unary operators are:
- Increment and Decrement Operators
Java also provides increment and decrement operators: ++ and -- respectively. ++ increases the value of the operand by 1 , while -- decrease it by 1 . For example,
Here, the value of num gets increased to 6 from its initial value of 5 .
Example 5: Increment and Decrement Operators
In the above program, we have used the ++ and -- operator as prefixes (++a, --b) . We can also use these operators as postfix (a++, b++) .
There is a slight difference when these operators are used as prefix versus when they are used as a postfix.
To learn more about these operators, visit increment and decrement operators .
6. Java Bitwise Operators
Bitwise operators in Java are used to perform operations on individual bits. For example,
Here, ~ is a bitwise operator. It inverts the value of each bit ( 0 to 1 and 1 to 0 ).
The various bitwise operators present in Java are:
These operators are not generally used in Java. To learn more, visit Java Bitwise and Bit Shift Operators .
Other operators
Besides these operators, there are other additional operators in Java.
The instanceof operator checks whether an object is an instanceof a particular class. For example,
Here, str is an instance of the String class. Hence, the instanceof operator returns true . To learn more, visit Java instanceof .
The ternary operator (conditional operator) is shorthand for the if-then-else statement. For example,
Here's how it works.
- If the Expression is true , expression1 is assigned to the variable .
- If the Expression is false , expression2 is assigned to the variable .
Let's see an example of a ternary operator.
In the above example, we have used the ternary operator to check if the year is a leap year or not. To learn more, visit the Java ternary operator .
Now that you know about Java operators, it's time to know about the order in which operators are evaluated. To learn more, visit Java Operator Precedence .
Table of Contents
- Introduction
- Java Arithmetic Operators
- Java Assignment Operators
- Java Relational Operators
- Java Logical Operators
- Java Unary Operators
- Java Bitwise Operators
Before we wrap up, let’s put your knowledge of Java Operators: Arithmetic, Relational, Logical and more to the test! Can you solve the following challenge?
Write a function to return the largest of two given numbers.
- Return the largest of two given numbers num1 and num2 .
- For example, if num1 = 4 and num2 = 5 , the expected output is 5 .
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
Java Tutorial
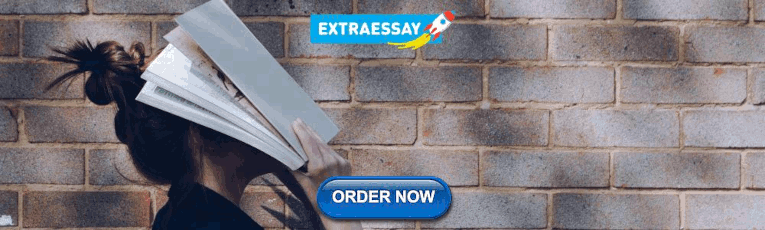
IMAGES
VIDEO